Bash scripting is a powerful tool for automating tasks on Linux and macOS systems. One of the fundamental building blocks for making decisions within your scripts is the if-else
statement. This statement allows your script to check conditions and execute different pieces of code based on whether those conditions are true or false.
In this blog post, we’ll delve into the world of if-else
statements in Bash. We’ll cover the basic syntax, explore how to use conditions, and demonstrate how to implement elif
statements for handling multiple scenarios. We’ll also touch on advanced techniques like combining conditions and using case
statements as an alternative.
What is an if else Statement?
An if else statement is a control structure that allows your Bash script to make decisions based on conditions. This means you can execute different pieces of code depending on whether a condition is true or false.
Imagine you have a script that needs to check if a file exists before proceeding. With an if else statement, you can elegantly handle this scenario, ensuring your script behaves correctly under various conditions.
Basic Syntax of if-else Statements
Here’s the basic syntax for an if-else
statement in Bash:
if [ condition ]
then
# code to execute if condition is true
else
# code to execute if condition is false
fi
The if Keyword
The if
keyword introduces a conditional statement. It tells the script to evaluate a given condition.
Conditions
Conditions are expressions that the script evaluates to determine if they are true or false. These expressions can involve:
Condition Type | Expression | Meaning |
---|---|---|
String Comparisons | = | Equals |
!= | Not equals | |
Numerical Comparisons | eq | Equals |
ne | Not equals | |
lt | Less than | |
le | Less than or equal to | |
gt | Greater than | |
ge | Greater than or equal to | |
File Tests | e | File exists |
f | File is a regular file | |
d | Directory exists |
The then Keyword
The then
keyword follows the condition and indicates the beginning of the code block that will execute if the condition is true.
The else Keyword
The else
clause adds a layer of complexity and flexibility to your Bash scripts by providing an alternative code block to execute when the if
condition is false. This ensures that your script can handle multiple scenarios gracefully.
The fi Keyword
The fi
keyword ends the if
statement. It signals the conclusion of the conditional structure.
How if-else Works
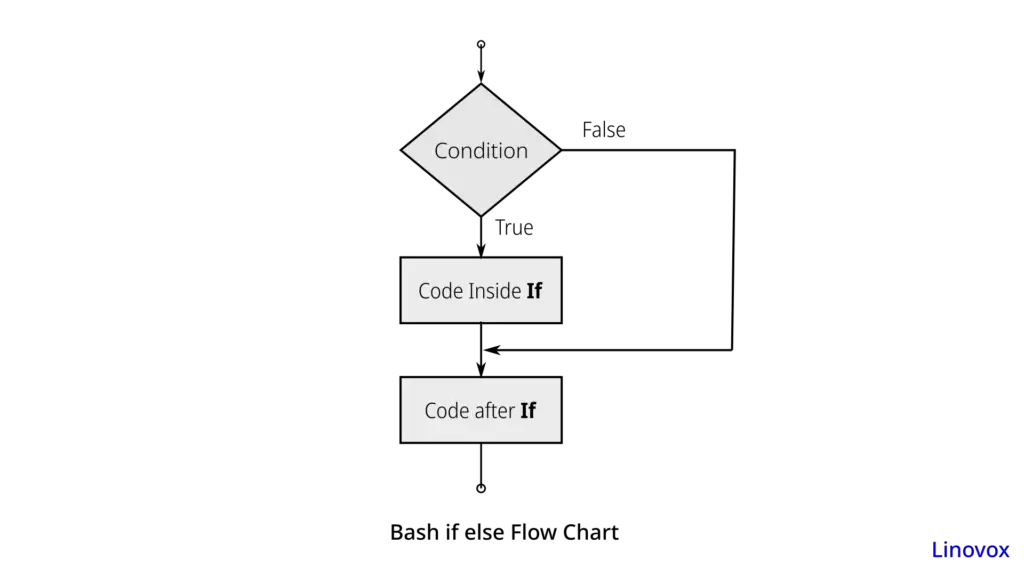
if
Keyword: Evaluates the condition.- Condition: The expression that is checked to be true or false.
then
Keyword: Starts the code block if the condition is true.else
Keyword: Introduces the alternative code block if the condition is false.fi
Keyword: Ends the conditional statement.
Example
Let’s look at a simple example where we check if a user is an admin:
user_role="admin"
if [ "$user_role" = "admin" ]
then
echo "Welcome, Admin!"
else
echo "Access denied."
fi
Adding elif for Multiple Conditions
The elif
(short for “else if”) clause further enhances the decision-making capabilities of Bash scripts by allowing you to check multiple conditions in sequence. This is especially useful when you have more than two possible outcomes for a given scenario.
Basic Syntax of if-elif-else Statements
Here’s the basic syntax for an if-elif-else
statement in Bash:
if [ condition1 ]
then
# code to execute if condition1 is true
elif [ condition2 ]
then
# code to execute if condition2 is true
else
# code to execute if neither condition1 nor condition2 is true
fi
How elif Works
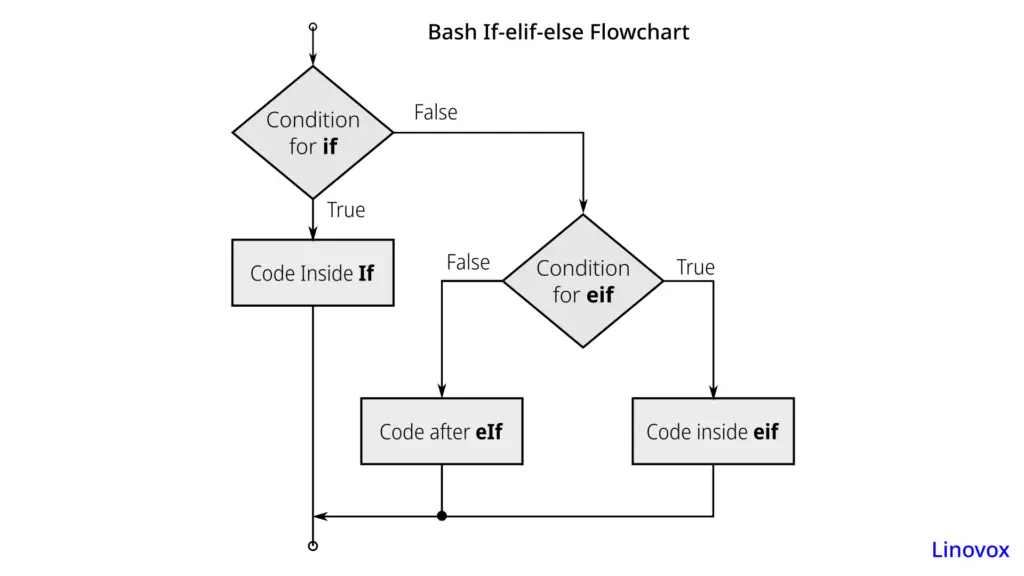
if
Keyword: Evaluates the first condition.elif
Keyword: Checks additional conditions if the first condition is false.else
Keyword: Provides a default action if none of the conditions are true.- Condition: The expression that is checked to be true or false.
then
Keyword: Starts the code block associated with the condition.fi
Keyword: Ends the conditional statement.
Simple Example
Let’s say we want to categorize a given number as positive, negative, or zero:
read -p "Enter a number: " number
if [ "$number" -gt 0 ]
then
echo "The number is positive."
elif [ "$number" -lt 0 ]
then
echo "The number is negative."
else
echo "The number is zero."
fi
Explanation of the Example
- Condition 1:
[ "$number" -gt 0 ]
checks if the number is greater than 0. - Condition 2:
[ "$number" -lt 0 ]
checks if the number is less than 0. - Else Block: Provides a default action if neither condition is true.
Example: Using elif for Multiple Conditions
Here’s another example that uses elif
to handle multiple conditions. We’ll create a script to assign grades based on a score:
#!/bin/bash
score=85
if [ $score -ge 90 ]; then
echo "Grade: A"
elif [ $score -ge 80 ]; then
echo "Grade: B"
elif [ $score -ge 70 ]; then
echo "Grade: C"
else
echo "Grade: F"
fi
- $score -ge 90: This condition checks if the score is greater than or equal to 90.
- elif: Allows checking additional conditions if the previous ones are false.
- else: Executes if none of the conditions are true.
Nested If Statements
Sometimes, you might need to check a condition only if a previous condition is true. This is where nested if statements come into play. Here’s an example:
#!/bin/bash
user="admin"
permission="read"
if [ $user == "admin" ]; then
if [ $permission == "read" ]; then
echo "Access granted."
else
echo "Access denied."
fi
else
echo "User not recognized."
fi
- Nested if: An if statement within another if statement to handle more complex logic.
Advanced If Else Statement
Now that we’ve covered the basics, it’s time to dive into some advanced techniques that will make your Bash scripts more powerful and efficient. In this section, we’ll explore combining conditions, using case statements as an alternative, and handling errors and debugging.
Combining Conditions
Sometimes, you need to check multiple conditions simultaneously. In Bash, you can combine conditions using logical operators such as AND (&&
) and OR (||
). This lets your Bash scripts handle intricate situations in a more concise way.
Example: Ensuring Multiple Conditions Are Met
Let’s write a script that checks if a user is eligible based on age and membership status:
#!/bin/bash
age=20
membership_status="active"
if [ $age -ge 18 ] && [ $membership_status == "active" ]; then
echo "You are eligible."
else
echo "You are not eligible."
fi
- &&: Logical AND operator ensures both conditions must be true.
- [ $age -ge 18 ]: Checks if age is 18 or older.
- [ $membership_status == “active” ]: Checks if the membership status is active.
Example: Checking Either Condition
Here’s a script that grants access if either of two conditions is met:
#!/bin/bash
role="admin"
access_level="read"
if [ $role == "admin" ] || [ $access_level == "write" ]; then
echo "Access granted."
else
echo "Access denied."
fi
- ||: Logical OR operator ensures only one of the conditions needs to be true.
- [ $role == “admin” ]: Checks if the user role is admin.
- [ $access_level == “write” ]: Checks if the access level is write.
Using Case Statements as an Alternative
For scripts that need to handle multiple discrete values, case
statements can be a more readable alternative to multiple elif
statements. They are especially useful for menu-driven scripts or when dealing with a fixed set of options.
Example: Menu Selection Script
Let’s create a simple menu selection script using a case
statement:
#!/bin/bash
echo "Select an option:"
echo "1) Start"
echo "2) Stop"
echo "3) Restart"
read option
case $option in
1)
echo "Starting the service..."
;;
2)
echo "Stopping the service..."
;;
3)
echo "Restarting the service..."
;;
*)
echo "Invalid option."
;;
esac
- case $option in: Begins the case statement.
- 1): Matches the value of
option
to 1. - ;;: Ends each case block.
- ): The default case if no other match is found.
Conclusion
We’ve explored the if-else
statement in Bash, a powerful tool for making decisions within your scripts. You’ve learned the basic syntax, how to craft conditions, and even tackled advanced techniques like combining conditions and using case
statements.
By now, you’re well-equipped to leverage if-else
statements to craft robust Bash scripts that can adapt to various conditions.