Are you looking to perform find and replace operations across documents? Vim is a powerful editor that allows you to find and replace text within entire documents and even across multiple documents simultaneously.
In this blog post, I’ll discuss the basic to advanced usage of the find and replace command in Vim, along with examples to help you better understand its functionality.
Table of Contents
Syntax of the Find and Replace Command
The basic syntax of the find and replace command in Vim is as follows:
:%s/{search_pattern}/{replacement_pattern}/{flags}
:%s/{search_pattern}/{replacement_pattern}/{flags}
- The
%
symbol indicates that the find and replace operation will be performed on the entire document. - The
s
character tells Vim to substitute the text. {search_pattern}
represents the text or pattern you want to find.{replacement_pattern}
specifies the text that will replace the found patterns.{flags}
are optional modifiers used to customize the find and replace command.
Vim Editor supports multiple flags to customize the find and replace operation according to your needs. You can also combine multiple flags to achieve your desired output. Here is a reference table of flags available in the Vim editor:
Flag | Description |
---|---|
g | Global replacement: Replaces all occurrences of the search pattern within a line. |
c | Confirmation prompt: Prompts for confirmation before each substitution. |
n | Dry run: Displays the number of matches without performing any substitutions. |
e | Suppress error: Suppresses the error message when a substitution fails. |
i | Case-insensitive matching: Ignores the case when matching the search pattern. |
I | Case-sensitive matching: Considers the case when matching the search pattern. |
p | Show replacements: Displays each replacement made in the quickfix window. |
s | Silent mode: Suppresses most of the normal output, only showing error messages. |
w | Whole word matching: Matches the search pattern only if it forms a whole word. |
& | Repeat last substitution: Repeats the last :substitute command. |
Simple Find and Replace Operations
Performing a find and replace operation once is the most fundamental use of the Vim find and replace command. In this section, we will start with finding and replacing a single occurrence up to multiple occurrences.
1. Finding and Replacing a Single Occurrence
To find and replace a single word in a document, first put the cursor on the line, and then use the following command:
:s/{search_pattern}/{replacement_pattern}
For example, if I have copied the GRUB configuration for this blog post and I want to change the kernel parameters from “loglevel=3” to “loglevel=0,” I can use the find and replace command in the following way:
:s/loglevel=3/loglevel=0
Vim will find the first occurrence of “loglevel=3” and replace it with “loglevel=0” within the current line.
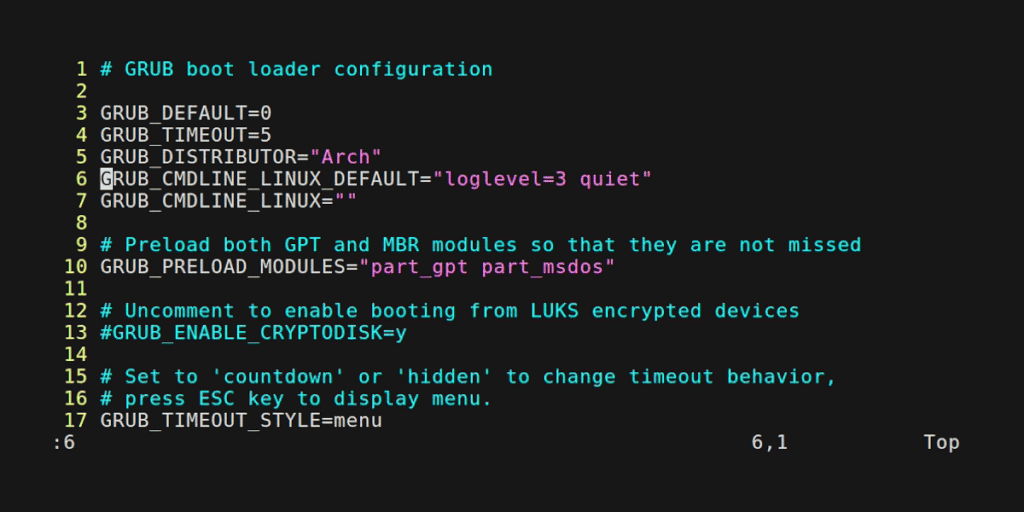
2. Finding and Replacing All Occurrences within a File
If you want to find and replace all occurrences within an entire file, you can do this by adding the global flag (g
) to the find and replace command.
:%s/{search_pattern}/{replacement_pattern}/g
For example, if you want to change every occurrence of “GRUB” to “Systemd” within the GRUB configuration file, you would use the following command:
:%s/GRUB/Systemd/g
Vim will replace all occurrences of “GRUB” with “Systemd” throughout the file.
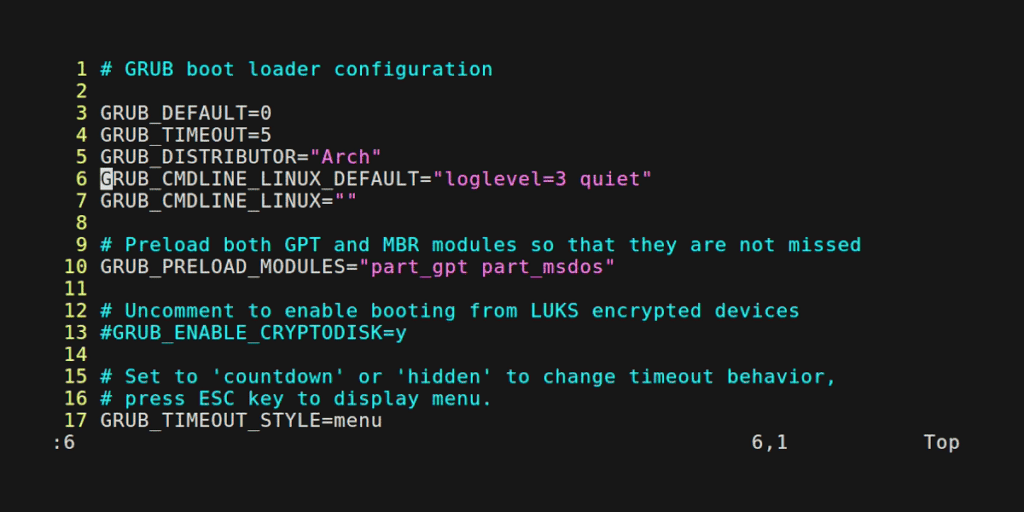
3. Highlighting Matches before Replacing
It is a good idea to highlight matches before making changes to reduce unwanted modifications. Vim supports highlighting match patterns, and you can enable this option with the hlsearch
option. You can toggle this option by entering the command:
:set hlsearch
After enabling hlsearch
, when you perform a find and replace operation, Vim will highlight all matches in the file, making it easier to verify the correctness of the replacements.
For our previous example, we can enable hlsearch
to see the match patterns and ensure that we are finding and replacing the correct word. In our previous example, I enable hlsearch
and then enter the search and replace command to replace all occurrences within the entire file. Vim highlights our match patterns within the entire directory.
:%s/GRUB/Systemd/g
By using this simple find and replace command, you can fulfill the basic needs of finding and replacing lines or words.
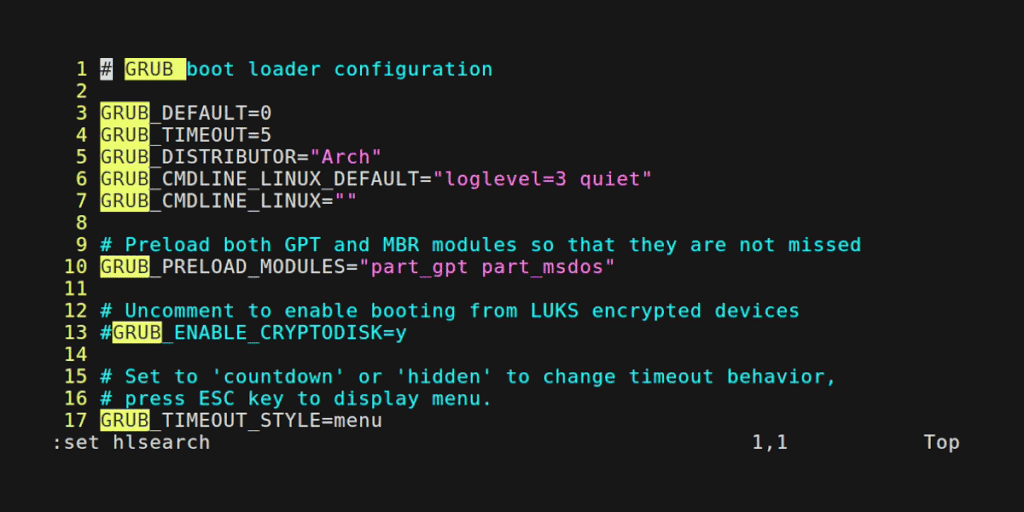
Find and Replace Using Regular Expressions (regex)
Regular expressions, also known as regex, are patterns that allow you to effectively search for text within large documents. You can combine regular expressions with Vim’s search and replace command to search for complex patterns in your documents and make changes with minimum effort.
For example, suppose you have a file with lines that start with the word “Error” followed by a specific error code. To find and replace these error codes, you can use the following regular expression:
:%s/Error \(\d\+\)/Replacement \1/g
In this example, the pattern Error \(\d\+\)
matches the word “Error” followed by one or more digits. The backreference \1
is used in the replacement pattern to retain the matched digits while replacing the entire match with the desired text.
Using Metacharacters for Advanced Matching
Metacharacters are special characters in regular expressions that have predefined meanings. Using metacharacters with Vim’s find and replace command allows for a more advanced way to search for a pattern. Here is a reference table of metacharacters and their descriptions:
Metacharacter | Description |
---|---|
. | Matches any single character except a newline. |
* | Matches zero or more occurrences of the preceding character or group. |
+ | Matches one or more occurrences of the preceding character or group. |
? | Matches zero or one occurrence of the preceding character or group. |
[] | Matches any single character within the brackets. |
() | Groups multiple characters or expressions together. |
For example, suppose you want to find and replace email addresses in a file. You can use the following regular expression to match email patterns:
:%s/[A-Za-z0-9._%+-]\+@[A-Za-z0-9.-]\+\.[A-Za-z]\{2,}/[email protected]/g
This regular expression matches a typical email pattern, such as `[email protected]. You can replace it with the desired text,
[email protected]`.
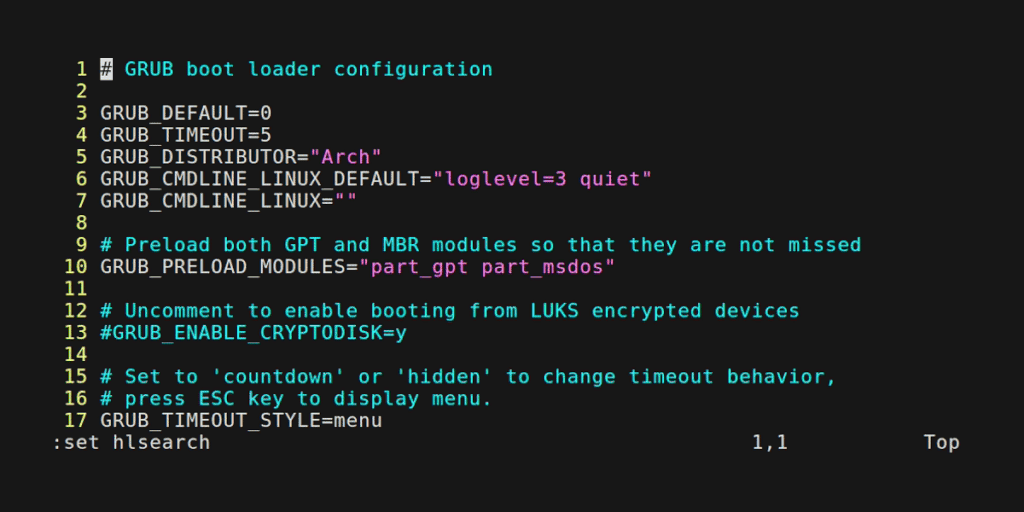
Leveraging Backreferences for Advanced Substitution
Backreferences allow you to capture and reuse portions of a matched pattern in the replacement. They are denoted by \
followed by a number. The number represents the order of the capturing group within the search pattern.
For instance, let’s say you have a file with lines that contain names in the format “last_name, first_name”. To swap the names into the format “first_name last_name”, you can use backreferences:
:%s/\(\w\+\), \(\w\+\)/\2 \1/g
In this example, the capturing groups \(\w\+\)
capture the last name and the first name separately. The backreferences \1
and \2
are used in the replacement pattern to swap the order of the names.
Find and Replace Across Multiple Files
Vim’s find and replace command works not only on a single document but also on multiple documents at a time, allowing you to find and replace with minimum effort. This option is useful when you work with a set of files that need consistent modifications.
1. Searching for Patterns in Multiple Files
To search for a specific pattern across multiple files, you can use Vim’s :vimgrep
command. This command allows you to search for a pattern in a specified set of files or within a directory.
The basic syntax for using :vimgrep
is as follows:
:vimgrep /{search_pattern}/ {file_pattern}
{search_pattern}
represents the pattern you want to find.{file_pattern}
specifies the files or directory where the search should be performed.
For example, if you want to search for the word “example” in all files with the .txt
extension within the current directory and its subdirectories, you would use the following command:
:vimgrep /example/ **/*.txt
Vim will display a list of matching lines and their corresponding file names in the quickfix window.
2. Performing Find and Replace Across Multiple Files
Once you have identified the files in which you want to perform find and replace operations, you can utilize Vim’s :argdo
command in combination with the :substitute
command to execute replacements across multiple files.
The basic syntax for using :argdo
and :substitute
together is as follows:
:argdo %s/{search_pattern}/{replacement_pattern}/g | update
{search_pattern}
represents the pattern you want to find.{replacement_pattern}
specifies the text that will replace the matched patterns.
For example, let’s say you want to replace the word “apple” with “orange” in all files with the .txt
extension within the current directory and its subdirectories. You would use the following command:
:argdo %s/apple/orange/g | update
Vim will iterate through each file in the argument list, perform the find and replace operation, and save the changes.
3. Prompting for Confirmation during Find and Replace
In situations where you want to review and confirm each replacement individually, you can use the c
flag in the :substitute
command. This flag prompts Vim to confirm each substitution.
The modified syntax for the find and replace command with confirmation is as follows:
:argdo %s/{search_pattern}/{replacement_pattern}/gc | update
– The `c` flag stands for confirmation.
For example, if you want to replace the word “foo” with “bar” in all files within the current directory and its subdirectories, prompting for confirmation before each substitution, you would use the following command:
:argdo %s/foo/bar/gc | update
Vim will prompt you for confirmation before performing each substitution. You can choose to replace the occurrence or skip it.
Keep in mind that when using :argdo
, Vim will open each file in a separate buffer. To navigate between buffers, you can use the :bn
command to switch to the next buffer or :bp
to switch to the previous buffer.
Conclusion
Vim’s find and replace command offers powerful capabilities for searching and replacing text within documents and across multiple files. By utilizing different flags, regular expressions, and backreferences, you can perform precise and efficient find and replace operations.